Send Push notifications to Phone in Python
Introduction
Sending push notifications to your phone can be a useful way to stay informed about important events or updates in your application, even when you’re away from your computer. In fact, we have used these to develop multiple Python projects and they always come in handy when developing.
Receiving notifications to your phone in Python can be useful in a variety of use cases. For example, if you have a script that runs periodically, such as a web scraper, you may want to be notified if there is an error or if the script has completed successfully. This way, you don’t have to constantly check the status of the script.
Another use case could be for monitoring systems or servers. For example, if you are running a website, you may want to be notified if your server’s memory or CPU usage exceeds a certain threshold. This way, you can take action to prevent a potential outage or performance issue.
Additionally, push notifications can be used for personal applications such as a reminder for your daily tasks or for keeping track of your stocks.
In short, receiving push notifications to your phone in Python can save you time and make it easier to stay informed and take action when necessary.
There are several ways to send push notifications to your phone using Python, from free to paid apps. In this article, we will be covering Pushover.
Pushover (one-time fee)
Pushover is a service that allows you to send push notifications to your iPhone, iPad, Android and even Desktop devices. It has a simple API that can be easily integrated into your Python application. We have been a happy user of them since 2020 and never encountered any issues.
They are available on multiple platforms, including:
Additionally, Pushover offers a variety of options for customizing the notification, such as adding an image or sound to the notification.
Pricing
At the time of writing, it is important to note that there are no subscription fees to Pushover which makes them a top choice for individual developers.
To use Pushover for yourself, it’s just a $5 USD one-time purchase on each platform (iPhone/iPad, Android, and Desktop) where you want to receive Pushover notifications.
Additionally, you can try it out free for 30 days by downloading the iPhone/iPad or Android apps. Multiple devices of the same platform are covered under the same one-time purchase, so you can use Pushover on your iPhone and iPad, or an Android phone and Android tablet without paying anything extra.
Please keep in mind that there is a 10,000 message per month limit on Pushover accounts. For my usecases, this was plenty but it is important to make sure that limit will work for your applications.
Getting started
Before following our tutorial, you will need to sign up for a Pushover account here. After signing-up and following the registration process, we recommend downloading the Pushover apps to your desired devices here:
Next, you will receive your User API key which can be found by logging into the Pushover dashboard. The user API key is used to identify your Pushover account, make sure not to share it with anyone.
It should look like this:
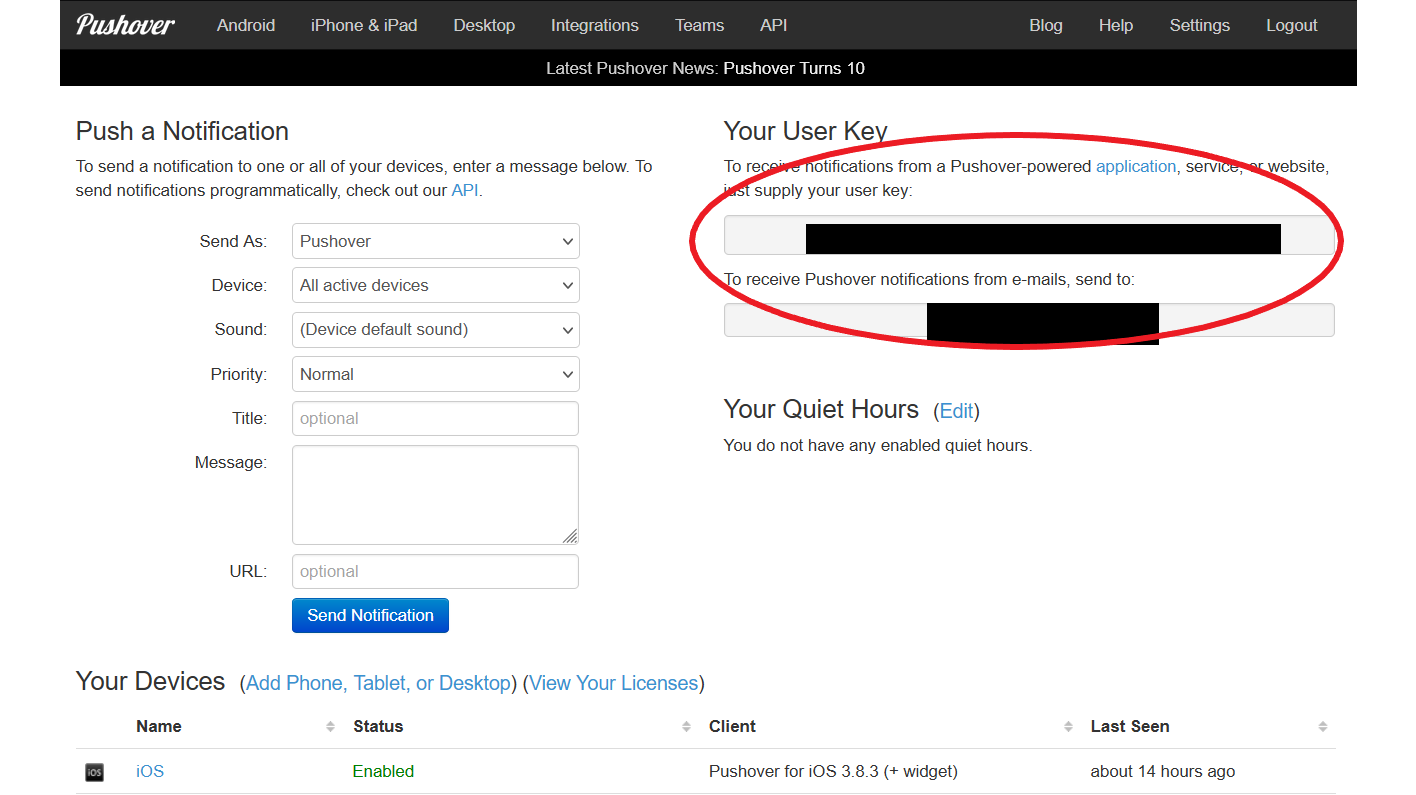
Now, you will need to create a new Application API token on the Pushover dashboard here.
It should look like this:
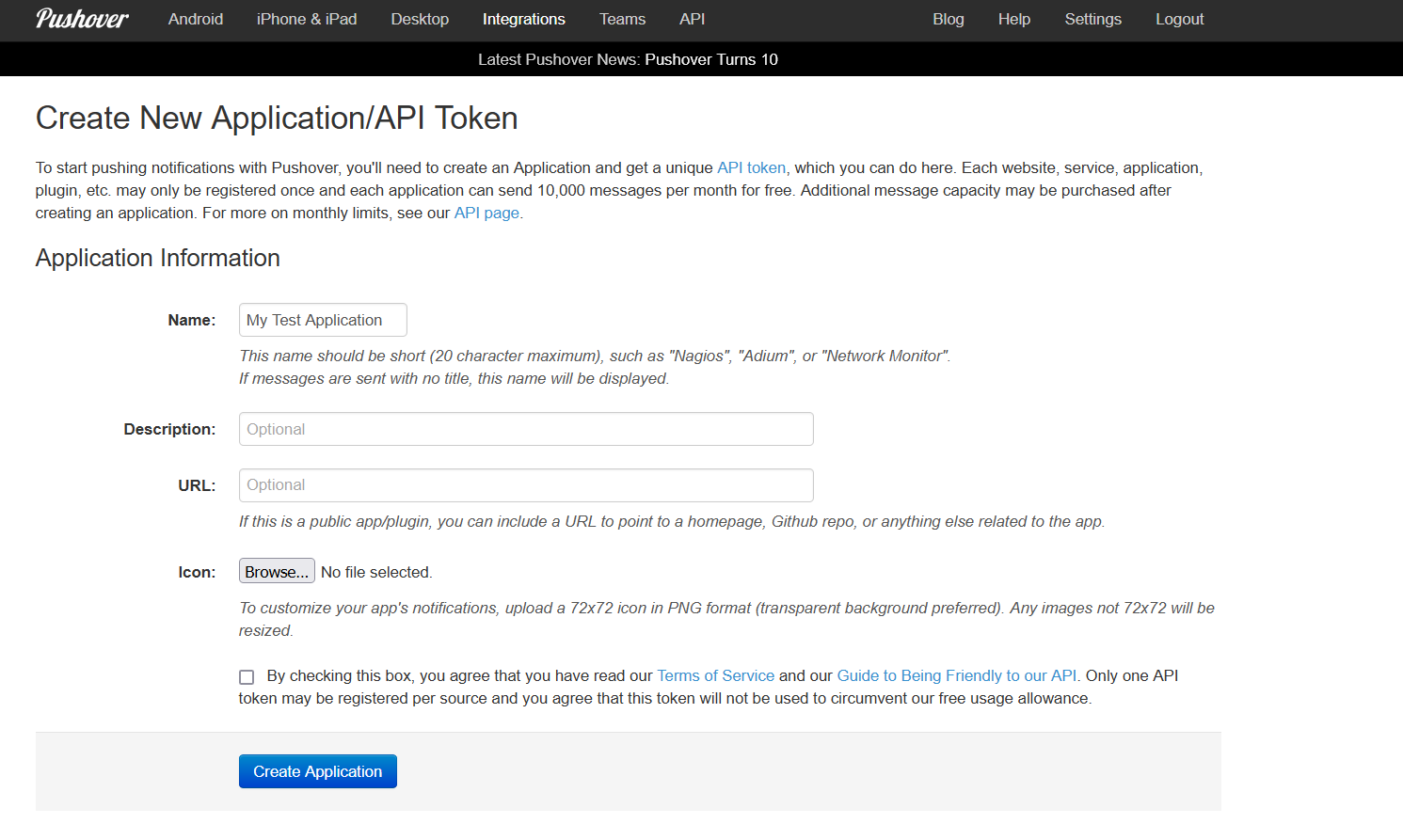
Finally, we should receive our Application API key after clicking on the Create application
button. Therefore, once we have our User API key as well as our Application API key, We are ready to start coding our Python script.
import requests
# Define your USER_TOKEN AND APPLICATION_TOKEN HERE:
APPLICATION_TOKEN = ...
USER_TOKEN = ...
message = "Hello world from Python!"
url = 'https://api.pushover.net/1/messages.json'
title = 'My message title'
my_pushover_request = {'token': APPLICATION_TOKEN, 'user': USER_TOKEN,
'title': title, 'message': message}
requests.post(url, data=my_pushover_request)
This Python code is using the requests library to send a push notification to a device using the Pushover service. First, the requests library is imported, which is used to send the post request to the Pushover API.
Then, two variables APPLICATION_TOKEN
and USER_TOKEN
are defined and initialized with the proper values (that you saved on the previous section). These are unique keys that are used to identify the application and the user account respectively.
A variable message
is defined and initialized with the string "Hello world from Python!"
which will be the message to be sent in the push notification.
A variable url
is defined and initialized with the endpoint of the Pushover API, which is the URL where the post request will be sent to.
A variable title
is defined and initialized with the string "My message title"
which will be the title of the push notification.
A variable my_pushover_request
is defined as a dictionary containing the required data to send the notification. This includes the token, user, title and message keys and the corresponding values.
Finally, the requests.post()
function is used to send a post request to the url endpoint with the my_pushover_request data.This sends the push notification to the registered device with the specified message and title. And, voila! you should’ve received the notification to your device which you installed the Pushover app on.
Note that the Pushover API offers many other features such as sending an image attachment, changing the message sound, etc. We recommend having a look to their API documentation for more information.
Conclusion
In conclusion, sending push notifications to your phone can be a useful way to stay informed about important events or updates in your application, even when you’re away from your computer. In this article, we have covered the use of Pushover, a service that allows you to send push notifications to your iPhone, iPad, Android and even Desktop devices.
The Pushover API is simple to integrate into your Python application and offers a variety of options for customizing the notification.
Pushover is a one-time purchase of $5 on each platform you want to receive notifications on and also comes with a 10,000 messages per month limit. The fact that it is a one-time purchase and that it works on multiple platforms makes it a great choice for individual developers and small projects.
However, Pushover is not the only service that allows you to send push notifications in Python. Other popular services include Firebase Cloud Messaging, OneSignal, among others. Each service has its own set of features and pricing plans, so it’s important to research and compare the different options to find the one that best suits your needs.